http://www.sparkfun.com/products/8883
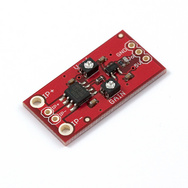
![]() |
  | |||||||||||||
![]() |
|
![]() |
||||||||||||
The Netduino forums have been replaced by new forums at community.wildernesslabs.co.
This site has been preserved for archival purposes only
and the ability to make new accounts or posts has been turned off.
Community Stats
#8554 Analog Read
Well I'm stumped. I don't think the netduino can measure current directly. You might need something like this that outputs varying voltage:
http://www.sparkfun.com/products/8883 ![]() #8121 How can I get instant (or close to) data from http...?
Not entirely sure I understand what you're looking for?
1. How to get your javascript to get live data w/o refreshing the whole page? 2. Or a different way to get data from the netduino? For #2, I don't know what you're going for. For #1, check out jquery and the load command: (this would go in your refresh function once you have jquery included) $('#myDiv').load('data.htm'); (to add jquery, use: <script type="text/javascript" src="http://code.jquery.com/jquery-latest.min.js"></script>in your <head>) ... in your program.cs: (i have not tested this, so it's more of an idea than anything) namespace NetduinoPlusWebServer { public class Program { public static void Main() { Listener webServer = new Listener(RequestReceived); OutputPort led = new OutputPort(Pins.ONBOARD_LED, false); while (true) { // Blink LED to show we're still responsive led.Write(!led.Read()); Thread.Sleep(500); } } private static void RequestReceived(Request request) { if (request.URL == "data.htm") { request.SendResponse("some kind of data"); } } } }
#7340 Analog in put C# coding
That's a cool little car. Is this all you're looking for, to test? Hook up 3.3v to aref, and the analog output from the sensor to A5 (plus whatever else the sensor needs, like power and ground), and i think you'll be all set to test.
using System.Text; using Microsoft.SPOT; using System.Threading; using SecretLabs.NETMF.Hardware; using SecretLabs.NETMF.Hardware.Netduino; namespace NDP_SocketSender1 { public class Program { public static void Main() { AnalogInput a5 = new AnalogInput(Pins.GPIO_PIN_A5); while (true) { string s = a5.Read().ToString(); Debug.Print(s); Thread.Sleep(100); } } } } The above code is from here.
#7053 StepGenie (An EE's Best friend?)
LOL... she was very grateful to receive a Google spreadsheet with part numbers from me this year. I was grateful to receive fewer sweaters. ![]() #7024 StepGenie (An EE's Best friend?)
Cool, thank you.
#7007 StepGenie (An EE's Best friend?)
I'm looking myself! My wife got me a bi-polar for Christmas, so... I either need to find a unipolar, or build the circuit to handle the bi-polar using a h-bridge.
#7000 StepGenie (An EE's Best friend?)
No, you'd hook a unipolar (with 6 wires) up to pins 5,6,7,8. The remaining two wires go to your powersource, possible through a power resistor first.
#6992 StepGenie (An EE's Best friend?)
for testing, i wouldn't put the modes on a digital IO yet, it would be one more complicating factor.
I think 4 wires means bipolar and it won't work (easily) with the step genie, you want a 6-lead.
http://www.probotix....ipolar_bipolar/
#6979 StepGenie (An EE's Best friend?)
I got a stepgenie for Christmas, my wife said it came quickly, and it was packed very well. Strange.
You can definitely use the digital ios to set the various values high or low (1 or 0).
To test it, i would start with LEDs. Tie pins 1,2,3 to +5v -- 13,14 to Gnd. 11 and 12 to digital ios, and 5,6,7,8 to leds through resistors and LEDs (no mosfets). Everything else (4,9,10) can be left open.
This should put you in "Hi-torque" mode.
Depending on pin 12 (DIR), if it is high or low, the lit leds should shift "up" or "down" when you toggle pin 11 (STEP). I didn't save my code, but i just toggled a digital io and paused 200ms so I could see it.
Then, I played with pins 2 and 3 to try out the different modes.
One lesson I learned (which was the point, really!) was the difference between unipolar and bi-polar steppers. I got a bi-polar stepper for Christmas, but the stepgenie is designed for unipolar...
#5962 Reading temperature from DS18B20 using OneWire
Using Peter H. Anderson's awesome RS232 - OneWire Controller I have managed to successfully read temperature using the Dallas DS18B20 one wire temperature sensor.
The DS18B20 is really awesome, seems very accurate and very responsive. Considering my struggles getting reliable readings from a thermistor, I am finally ![]() Here is my temperature reading code, and attached is a class I wrote to interface with the OneWire controller, in case someone finds it useful. using System; using System.IO.Ports; using System.Text; using System.Threading; using Microsoft.SPOT; using Microsoft.SPOT.Hardware; using PHAOneWire; using SecretLabs.NETMF.Hardware; using SecretLabs.NETMF.Hardware.Netduino; namespace SerialTest1 { public class Program { public static void Main() { int Tc, Tf; using (OneWire ow = new OneWire()) { while (true) { ow.Write("P0W0ccS044"); Thread.Sleep(1000); // need to wait for conversion ow.Write("P0W0ccW0be"); byte low = ow.ReadByte(); byte high = ow.ReadByte(); // it's dealing with temps as integers, for instance, 23.5F comes back 235000 // since it's 12bits of precision, the smallest difference in temp is .0625 Tc = ((high * 256 + low) * 10000) / 16; Tf = ((Tc * 18) / 10) + 320000; Debug.Print(Tf.ToString() + " - " + Tc.ToString()); Thread.Sleep(10); } } } } } Attached Files
#4547 MFToolkit - new release (VS2010 support / .NET MF 4.1)
Is there a link to just what exactly the above MFToolkit is? I was not able to find a site that stated a simple overview of what it is and why it exists. Thanks!
| ||||||||||||||
![]() |
||||||||||||||
![]() |
|
![]() |
||||||||||||
![]() |
This webpage is licensed under a Creative Commons Attribution-ShareAlike License. | ![]() |
||||||||||||
![]() |