This is my first topic and project for Netduino platform. I still don't have mine yet, UPS bastards missed-up the shipment ):
I thought I could start programming and I tried to make EMF Detector similar to the one for Arduino by: Aaron ALAI’s EMF detector project.
I'm not sure if it's working correctly and I hope if anyone kindly could check it.
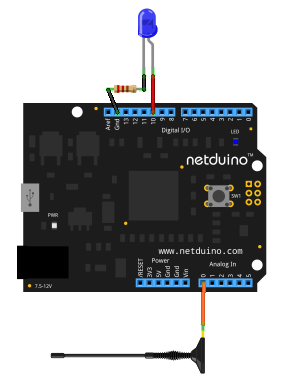
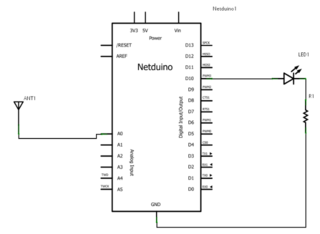
using System; using System.Threading; using Microsoft.SPOT; using Microsoft.SPOT.Hardware; using SecretLabs.NETMF.Hardware; using SecretLabs.NETMF.Hardware.NetduinoPlus; namespace NetduinoPlus_EMF_Detector { /// <summary>Original Project: Aaron ALAI’s EMF detector project for the Arduino.</summary> /// <remarks> http://www.aaronalai.com/emf-detector </remarks> public class Program { public static void Main() { const uint SAMPLES = 300; // No. of samples the device takes per reading. AnalogInput analogInput = new AnalogInput(Pins.GPIO_PIN_A0); // Assign analog PIN A0 as input port. PWM led = new PWM(Pins.GPIO_PIN_D10); // Assign GPIO PIN D10 as LED output port. uint dutyCycle = 0; // LED 8-bits output value: 0-255 (always off)-(always on). Default OFF. float avarage = 0.00f; // var holding the avarage of all samples. ulong sum = 0L; // var holding the sum of all samples. int[] readings = new int[SAMPLES]; // array holding actual samples. while (true) // loop infinitely { for (uint i = 0; i < SAMPLES; i++) { readings[i] = analogInput.Read(); // read data from the antenna wire. Thread.Sleep(10); // allow system to complete proccessing. sum += (ulong)readings[i]; // calculate sum. } avarage = sum / SAMPLES; // calculate the avarage of all samples. dutyCycle = (uint)constrain((long)avarage, 0, 100); // constrains the avarage value to between two numbers 0 and 100 dutyCycle = (uint)map((long)avarage, 0, 100, 0, 255); // maps out 0-100 to 0-255, 255 is the threshold of analog to digital conversion. led.SetDutyCycle(dutyCycle); // set the duty cycle value of LED. Thread.Sleep(10); // allow system to complete proccessing. // clean-up dutyCycle = 0; avarage = 0.00f; sum = 0L; } } /// <summary> /// Constrains a number to be within a range. /// </summary> /// <param name="value">the number to constrain, long data types.</param> /// <param name="low">the lower end of the range, long data types.</param> /// <param name="high">the upper end of the range, long data types.</param> /// <returns> /// <para>value: if value is between low and high.</para> /// <para>low: if value is less than low.</para> /// <para>high: if value is greater than high.</para> /// </returns> /// <example> /// <para>val = constrain(val, 0, 100);</para> /// <para>// limits range of val to between 0 and 100.</para> /// </example> /// <remarks> /// See: http://arduino.cc/en/Reference/Constrain /// </remarks> public static long constrain(long value, long low, long high) { return value >= high ? high : value <= low ? low : value; } /// <summary> /// Re-maps a number from one range to another. /// </summary> /// <param name="value">x: the number to map, long data types.</param> /// <param name="fromLow">the lower bound of the value's current range, long data types.</param> /// <param name="fromHigh">the upper bound of the value's current range, long data types.</param> /// <param name="toLow">the lower bound of the value's target range, long data types.</param> /// <param name="toHigh">the upper bound of the value's target range, long data types.</param> /// <returns> /// The mapped value. /// </returns> /// <remarks> /// See: http://arduino.cc/en/Reference/map /// </remarks> public static long map(long value, long fromLow, long fromHigh, long toLow, long toHigh) { try { return (value - fromLow) * (toHigh - toLow) / (fromHigh - fromLow) + toLow; } catch (Exception ex) { throw ex; } } } }